Grunt, Sass, Compass, Jekyll
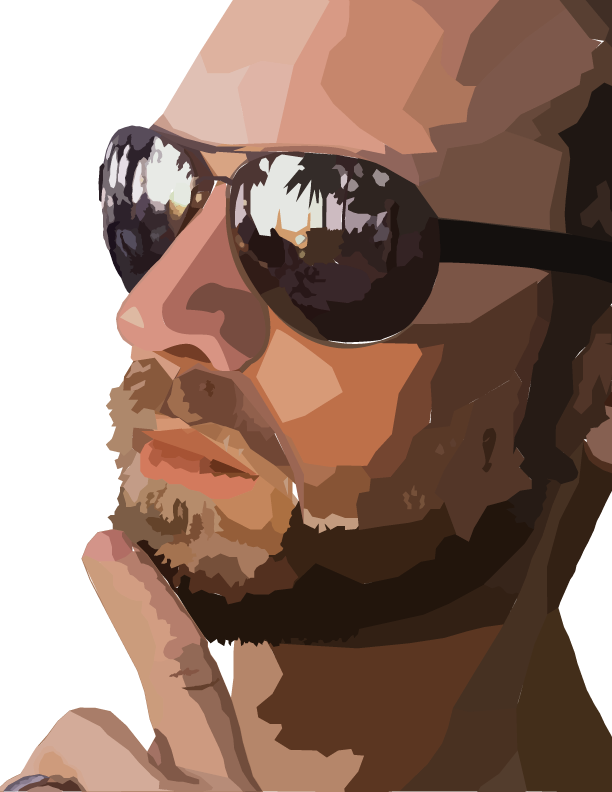
Jekyll
So I started my Jekyll project with playing around with the basic features set of how Jekyll handles templating. Once I had a good grasp on how the templating engine in Jekyll worked I really wanted to understand how the video in class utilized Jekyll Grunt and Sass. Since these three separate parts I decided to tackle them one at a time. I felt like Jekyll was the first one.
Jekyll Folder structure:-- _config.yml
-- _drafts
-- draft1.html
-- draft2.markdown
--_includes
-- footer.html
-- header.html
--_layouts
-- default.html
-- post.html
--_posts
-- 2014-3-5-Jeffs-thoughts-on-Jekyll.html
--_data
-- members.yml
--_site
--index.html
I was Playing with the templaing as I said earlier and the way that we use these files. _config.yml is used to store configuration data similarly to the way that package.json works with grunt installing and configuring grunt for you before the project is started.. this enables developers to work together as a team and have a specific configuration pre set up for them when they take off running.
As I had said the config file can have actually configuration setup in it to make Jekyll operate in a specific way currently all I have setup is for it to populate the header with a Title and the foote to populate with my contact information.
Grunt
I will be honest at this point because I was understanding how jekyll as a local server was working my curiosity of grunt took over at this point and I started play with grunt. After a few google searches I had a pretty good idea of what to do and I created my very first gruntfile.js and used node to configure grunt to work in this directory.
I started by creating a package.json file that installed grunt from npm (node package manager).
The Package.json File:
{
"name" : "portfolio",
"version" : "0.0.1",
"dependencies" : {
"grunt" : "~0.4.1",
"grunt-contrib-watch" : "~0.5.3",
"grunt-contrib-compass" : "~0.5.0",
"grunt-contrib-uglify" : "~0.2.2",
"matchdep" : "~0.1.2"
}// dependencies
}
So if you look at the above json The first bit should be rather self explanatory but all I am doing is giving my project a name and version number then things get a little bit more interesting with the dependencies.
Grunt is a is a javascript automated task runner.. Super cool
So essentially what I am doing is installing grunt and a few grunt plugins. it's the name of the program on the left and it corresponding version number on the right just to be clear.
Grunt Watch
What grunt watch does is almost exactly the same thing that jekyll does with automating post within jekyll. However, grunt also has this additional feature called live reload which during the dev process will force the browser to reload on any change.
This means that if you were to say change the background color variable in Sass as you hit save in your IDE the browser window will auto update. of course you have to configure grunt to "Watch" for sass changes which I will explain in a moment but in a nutshell this tool is super powerful
Here is the grunt configuration file gruntfile.js :
module.exports = function(grunt){
grunt.loadNpmTasks('grunt-contrib-uglify');
grunt.loadNpmTasks('grunt-contrib-watch');
grunt.loadNpmTasks('grunt-contrib-compass');
grunt.initConfig({
uglify: {
my_target: {
files: {
'_/js/main.js': ['_/components/js/*.js']
}//files
}//my_target
},//uglify
compass: {
dev: {
options: {
config: 'config.rb'
}//options
}//dev
},//compass
watch:{
options: { livereload: true },
script: {
files: ['_/components/js/*.js'],
tasks: ['uglify']
},//script
sass: {
files: ['_/components/sass/*.scss'],
tasks: ['compass:dev']
},//sass
html: {
files: ['*.html']
}//html
}//watch
})//initConfig
grunt.registerTask('default', 'watch');
}//exports
In the above grunt file we have a lot going on in here. First module.exports is a node.js command to install or load node package manager tasks. Essentially we are declaring module.exports a function quite simply.
Then we are loading uglify first which is just simply a compressor for javascript. It will compress multiple js files into a single javascript file similar to sass being compiled into css.
Then we load watch which like jekyll as I explained above will detect changes in the site.
then we have compass which is the engine that not only changes sass to css but depending on how you handle the out put could make it compressed for the sake of speed. Compass is actually a ruby gem that gets installed on the system and configured with a config.rb file which is ruby a language that to be honest I dont really know but I was able to look at the file and figure out how it works.
require "susy"
css_dir = '_/css'
sass_dir = '_/components/sass'
javascripts_dir = '_/js'
output_style = :compressed